Upgrade from Options API to Composition API with Vue 3 Script Setup
web-development
vuejs
javascript
frontend-development
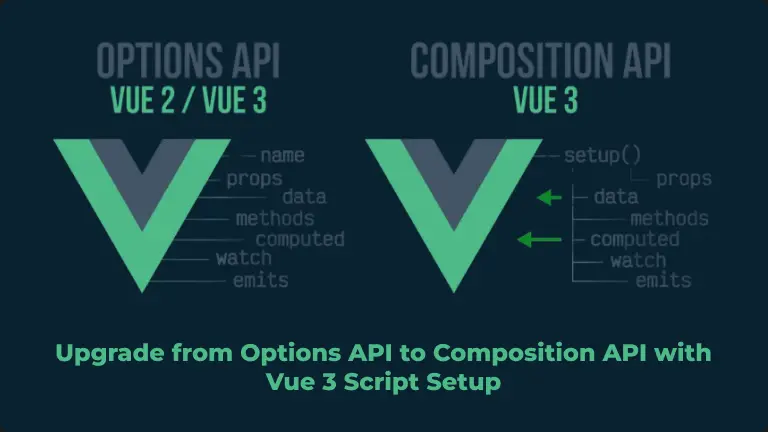
Streamline Your Vue 3 Development with <script setup>
With the release of Vue 3, the new <script setup>
syntax has revolutionized Vue component development. This magical feature simplifies your code by eliminating the need for export default
and the traditional setup()
function.
Why Use <script setup>
?
The <script setup>
syntax offers numerous benefits:
- Leaner Codebase: Reduce boilerplate and streamline your Vue components.
- Faster Development: Accelerate your development process with a more intuitive approach.
- Readable and Simplified Code: Condense component options into a single block for better clarity.
- Enhanced Reactivity: Make reactive programming more accessible and straightforward.
How to Use Props with <script setup>
In <script setup>
, you use the defineProps()
macro to handle props. This macro is available automatically and doesn't require any imports.
1<template>2 <div>3 <p>Received Prop: {{ propValue }}</p>4 </div>5</template>67<script setup>8const props = defineProps({9 propValue: String10});11</script>12
Methods in Composition API’s <script setup>
With <script setup>
, methods are no longer defined within an object. Instead, you declare functions as const
variables, which can be directly used in your template.
Options API:
1<template>2 <div>3 <button @click="incrementCounter">Increment</button>4 <p>Counter: {{ counter }}</p>5 </div>6</template>78<script>9export default {10 data() {11 return {12 counter: 013 };14 },15 methods: {16 incrementCounter() {17 this.counter++;18 }19 }20};21</script>22
Composition API:
1<template>2 <div>3 <button @click="incrementCounter">Increment</button>4 <p>Counter: {{ counter }}</p>5 </div>6</template>78<script setup>9import { ref } from 'vue';1011const counter = ref(0);1213const incrementCounter = () => {14 counter.value++;15};16</script>17
Using Computed Values in Composition API
In Composition API, computed values are similar to reactive data variables. They function as functions that return values based on reactive dependencies.
Options API:
1<template>2 <div>3 <button @click="incrementCounter">Increment</button>4 <p>Counter: {{ counter }}</p>5 <p>Double Counter: {{ doubleCounter }}</p>6 </div>7</template>89<script>10export default {11 data() {12 return {13 counter: 014 };15 },16 computed: {17 doubleCounter() {18 return this.counter * 2;19 }20 },21 methods: {22 incrementCounter() {23 this.counter++;24 }25 }26};27</script>28
Composition API:
1<template>2 <div>3 <button @click="incrementCounter">Increment</button>4 <p>Counter: {{ counter }}</p>5 <p>Double Counter: {{ doubleCounter }}</p>6 </div>7</template>89<script setup>10import { ref, computed } from 'vue';1112const counter = ref(0);1314const doubleCounter = computed(() => {15 return counter.value * 2;16});17</script>18