Vue.js Directives
web-development
vuejs
javascript
frontend-development
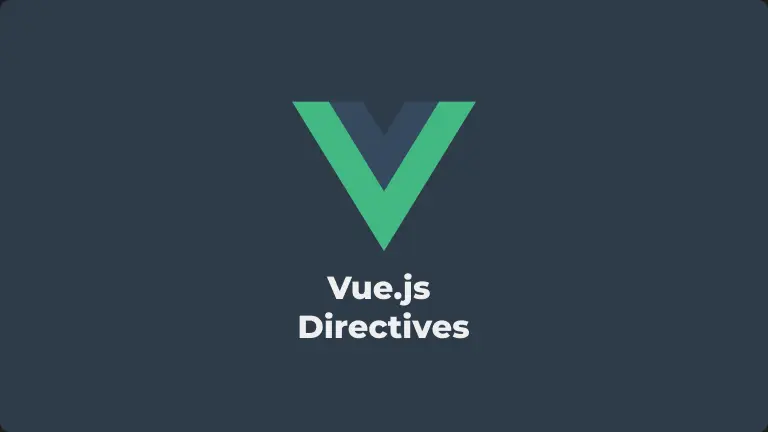
Introduction
Vue.js directives are essential tools for building dynamic web applications. Vue 3 provides a powerful set of directives for DOM manipulation and creating interactive user interfaces, making it a cornerstone of Vue.js development.
What Are Vue 3 Directives?
Vue 3 directives, easily identified by their "v-" prefix, are special attributes that extend the behavior of HTML elements. Understanding Vue 3 directives is crucial for effective Vue.js development. v-model Directive: Two-Way Data Binding The v-model directive is key for Vue 3 form handling, enabling two-way data binding on form inputs.
1<template>2 <input v-model="message" placeholder="Edit me" />3 <p>Message is: {{ message }}</p>4</template>56<script>7export default {8 data() {9 return {10 message: "",11 };12 },13};14</script>15
v-for Directive: Efficient List Rendering
The v-for directive is central to Vue 3 list rendering, allowing you to iterate over arrays and objects in your templates.
1<template>2 <ul>3 <li v-for="item in items" :key="item.id">4 {{ item.name }}5 </li>6 </ul>7</template>89<script>10export default {11 data() {12 return {13 items: [14 { id: 1, name: 'Item 1' },15 { id: 2, name: 'Item 2' },16 { id: 3, name: 'Item 3' }17 ]18 }19 }20}21</script>22
Conditional Rendering with v-if, v-else, and v-else-if
Mastering conditional rendering in Vue 3 with v-if, v-else, and v-else-if directives is crucial for creating dynamic UIs.
1<template>2 <div v-if="type === 'A'">3 Type A4 </div>5 <div v-else-if="type === 'B'">6 Type B7 </div>8 <div v-else>9 Not A or B10 </div>11</template>1213<script>14export default {15 data() {16 return {17 type: 'A'18 }19 }20}21</script>2223
v-bind and Shorthand Bindings: Dynamic Attribute Binding
The v-bind directive, essential for Vue 3 attribute binding, allows for dynamic HTML attributes in your applications.
12<template>3 <!-- Full syntax -->4 <img v-bind:src="imageSrc">56 <!-- Shorthand syntax -->7 <button :disabled="isButtonDisabled">Click me</button>8</template>910<script>11export default {12 data() {13 return {14 imageSrc: 'path/to/image.jpg',15 isButtonDisabled: false16 }17 }18}19</script>20
Custom Directives in Vue 3: Advanced Vue.js Techniques
Creating custom directives in Vue 3 is an advanced Vue.js technique that allows for unique DOM manipulations.
1// Global custom directive2app.directive('focus', {3 mounted(el) {4 el.focus()5 }6})78// Usage in component9<input v-focus>10
Best Practices for Using Vue 3 Directives
Optimizing Vue directives and following Vue 3 best practices are crucial for maintaining high Vue.js performance.
Use directives judiciously to avoid overuse Leverage directive modifiers for common scenarios Prioritize computed properties or methods for complex logic Always use the key attribute with v-for for optimal rendering Choose v-show over v-if for frequently toggled elements
Conclusion
Mastering Vue 3 directives is key to becoming proficient in Vue.js development. By understanding and effectively using these directives, you can create more efficient, reactive user interfaces and improve overall Vue.js performance. For a deeper dive into Vue 3 directives and to further your Vue.js learning, refer to the official Vue.js documentation. This revised version incorporates more of the identified keywords throughout the content, enhancing its potential for better search visibility and reach. The structure remains intact while emphasizing key Vue 3 concepts and best practices.